티스토리 뷰
Life is too short, You need python.
파이썬 기초 정리 2탄.
오늘은 파이썬의 대표적인 자료형을 정리해보고자 한다. (긴 글 주의.)
- 자료형 - 리스트, 튜플, 딕셔너리, 집합
원래 제어문과 예외처리 까지 하려고 했으나 너무 길어져서 실패.ㅠㅠ
초기 기획은 4탄까지 인데, 아마 4탄으로 안끝나지 싶다
이들 자료형은 컴퓨터 구조에서 정의하는 특정 자료구조들의 구현체이고 다음과 같은 특징을 가진다.
순서 | 수정 가능 여부 | 중복 가능 여부 | |
list [] | Ordered (O) | mutable (O) | duplicatable (O) |
tuple () | Ordered (O) | immutable (X) | duplicatable (O) |
dictionary {} | Unordered (X) | mutable (O) | non-duplicate (X) |
set {} | Unordered (X) | mutable (O) | non-duplicate (X) |
[2] - 4. 리스트 (List)
1. 선언
a = list()
a = []
#동일하게 빈 리스트를 선언한다.
파이썬은 동적 타입 언어이므로 하나의 리스트에도 여러 가지 자료를 담을 수 있다.
b= ["a",1,[1,2]]
2. 인덱싱
문자열과 마찬가지로 인덱싱 가능하다 0부터 시작 맨뒤의 항목은 -1부터 접근 가능
3. 연산
연산자 | 예시 | |
슬라이싱 | list[start:count] | >>> a = [1, 2, 3, 4, 5] >>> a[0:2] [1, 2] |
길이 구하기 | len() | >>> a = [1, 2, 3] >>> len(a) |
더하기 | + | >>> b = [4, 5, 6] >>> a + b [1, 2, 3, 4, 5, 6] |
반복하기 | * | a * 3 [1, 2, 3, 1, 2, 3, 1, 2, 3) |
수정 | list[index] = 2 | >>> a = [1, 2, 3] >>> a[2] = 4 >>> a [1, 2, 4] |
삭제 | del list[index] | >>> del a[1] [1, 4] |
4. 더 많은 연산
함수 | 비고 | |
append | list.append(x) | item 붙이기 |
extend | list.extend(iterable) | iterable 붙이기 |
insert | list.insert(i, x) | 특정 위치 삽입 |
remove | list.remove(x) | 삭제 - 해당 아이템이 없을 때 ValueError |
Pop | list.pop([i]) | 마지막 item 제거 |
clear | list.clear() | 모든 item 삭제 |
index | list.index(x[, start[, end]]) | x의 첫번째 인덱스 반환. - 해당 아이템이 없을 때 ValueError |
count | list.count(x) | x의 갯수 |
sort | list.sort(*, key=None, reverse=False) | 해당 목록을 정렬 *cf) sorted(iterable) 새로운 정렬된 목록을 반환 |
reverse | list.reverse() | *cf) reversed(iterable) 새로운 반되로 된 목록을 반환 |
copy | list.copy() | shallow 카피 a[:]와 동일 |
[2] - 5. 튜플 (Tuple)
1. 선언
>>> t1 = ()
>>> t2 = (1,) #하나만 선언할때는 반드시 콤마(,)를 붙여야 한다.
>>> t3 = (1, 2)
>>> t4 = 1, 2, 3 #괄호를 생략해도 무방하다.
리스트와 튜플이 다른 점은 삭제와 수정이 안 된다
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object doesn't support item deletion
2. 인덱싱
인덱싱은 리스트와 동일하다.
3. 연산
사용법은 리스트 참고
연산자 | |
슬라이싱하기 | tuple[start:count] |
길이 구하기 | len() |
더하기 | + |
곱하기 | * |
[2] - 6 딕셔너리 ( Dictionary)
Key와 Value를 한 쌍으로 갖는 자료형
1. 선언
>>> dic = {'name':'pey', 'phone':'0119993323', 'birth': '1118'}
>>> a = {1: 'hi'}
>>> a = { 'a': [1,2,3]}
2. 접근
딕셔너리는 인덱스로 접근하지 않고 오로지 키로 접근하는 것이 다른 자료구조와의 차이점이다.
>>> a = {1:'a', 2:'b'}
>>> a[1]
'a'
>>> a[2]
'b'
* 존재하지 않는 키를 찾을 때 다음과 같이 오류를 발생시킨다. 이후 등장하는 get함수와 다르다
>>> a = {'name':'pey', 'phone':'0119993323', 'birth': '1118'}
>>> print(a['nokey'])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'nokey'
3. 연산
연산 | 의미 |
a['name'] = 'pey' | 추가 또는 수정 |
del a['name'] | 삭제 |
4. 그 밖의 함수
의미 | 예시 | |
a.keys() | key list 반환 | >>> a = {'name': 'pey', 'phone': '0119993323', 'birth': '1118'} >>> a.keys() dict_keys(['name', 'phone', 'birth']) |
a.values() | value list 반환 | >>> a.values() dict_values(['pey', '0119993323', '1118']) |
a.items() | key, value 쌍(tuple) 반환 | >>> a.items() dict_items([('name', 'pey'), ('phone', '0119993323'), ('birth', '1118')]) |
a.clear() | 모든 항목 삭제 | 생략 |
a.gey(key) | key에 해당하는 Value 가져오기 * 존재하지 않는 Key의 경우 None 반환 |
>>> a.get('foo', 'bar') 'bar' |
"key" in a | 해당 Key가 있으면 True 그렇지 않으면 False |
>>> a = {'name':'pey', 'phone':'0119993323', 'birth': '1118'} >>> 'name' in a True >>> 'email' in a False |
[2] - 7. 집합 (Set)
1. 선언
>>> s1 = set([1,2,3])
>>> s1
{1, 2, 3}
집합 자료형은 중복을 허용하지 않고, 순서가 없다. 따라서 인덱싱 불가하다
2. 연산
연산 | 의미 |
s1 | s2 또는 s1.union(s2) |
합집합 |
s1 - s2 또는 s1.difference(s2) |
교집합 |
s1 & s2 또는 s1.intersection(s2) |
차집합 |
s1.add(4) | 항목 추가 |
s1.update([4, 5, 6]) | 여러개의 항목 추가 |
s1.remove(2) | 항목 삭제 |
드디어 길고 긴 포스팅이 끝난다.
수고했다 나자신.
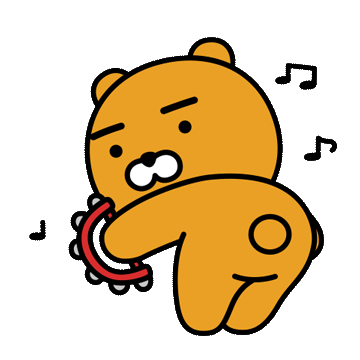
참고
사실 이것만 보면 됨
https://docs.python.org/3/tutorial/datastructures.html
5. Data Structures — Python 3.10.7 documentation
5. Data Structures This chapter describes some things you’ve learned about already in more detail, and adds some new things as well. 5.1. More on Lists The list data type has some more methods. Here are all of the methods of list objects: list.append(x)
docs.python.org
a['name'] = 'pey'
'Computer Engineering > Python' 카테고리의 다른 글
Python 기초 (1/4) - 점프 투 파이썬 (기본 자료형) (0) | 2022.05.16 |
---|